本文主要已代码的方式来讲述如何使用 Go
的 time
包文章源自编程技术分享-https://mervyn.life/c3ee5e76.html
package main
import (
"fmt"
"time"
)
func datetimeDemo() {
fmt.Printf("当前年份:%d\n", time.Now().Year())
fmt.Printf("当前月份:%d\n", time.Now().Month())
fmt.Printf("当前日子:%d\n", time.Now().Day())
fmt.Printf("当前小时:%d\n", time.Now().Hour())
fmt.Printf("当前分钟:%d\n", time.Now().Minute())
fmt.Printf("当前秒:%d\n", time.Now().Second())
now := time.Now()
//当前时间的时间戳
fmt.Printf("当前时间戳:%d\n", now.Unix())
//日期格式化
//YYYY (4位数表示完整年份)
fmt.Printf("当前的4位数年份为:%s\n", now.Format("2006"))
//yy (2位数表示的年份)
fmt.Printf("当前的2位数年份为:%s\n", now.Format("06"))
year, _, _ := time.Now().Date()
fmt.Printf("当前年份为:%d\n", year)
//mm 有前导零的月份
fmt.Printf("当前月份:%s月\n", now.Format("01"))
//n 没有前导零的月份
fmt.Printf("当前月份:%s月\n", now.Format("1"))
_, month, _ := time.Now().Date()
fmt.Printf("当前月份:%d月\n", month)
fmt.Printf("当前月份:%s\n", month)
//dd 月份中的第几天,有前导零的 2 位数字
fmt.Printf("当前日期:%s日\n", now.Format("02"))
//j 月份中的第几天,没有前导零
fmt.Printf("当前日期:%s日\n", now.Format("2"))
_, _, day := time.Now().Date()
fmt.Printf("当前月份:%d日\n", day)
//D 星期中的第几天,文本表示,3个字母
fmt.Printf("当前时间:%s\n", now.Format("Mon"))
//l 星期几,完整的文本格式
fmt.Printf("当前时间:%s\n", now.Format("Monday"))
//功能同上
fmt.Printf("当前时间:%s\n", now.Weekday().String())
//HH (24小时制)
fmt.Printf("当前时间:%s时\n", now.Format("15"))
//hh (12小时制,有前导零)
fmt.Printf("当前时间:%s时\n", now.Format("03"))
//g (12小时制,没有前导零)
fmt.Printf("当前时间:%s时\n", now.Format("3"))
fmt.Printf("当前时间:%d时\n", now.Hour())
hour, _, _ := now.Clock()
fmt.Printf("当前时间:%d时\n", hour)
//ii (有前导零的分钟数)
fmt.Printf("当前时间:%s分\n", now.Format("04"))
// (没有前导零的分钟数)
fmt.Printf("当前时间:%s分\n", now.Format("4"))
fmt.Printf("当前时间:%d分\n", now.Minute())
_, minute, _ := now.Clock()
fmt.Printf("当前时间:%d分\n", minute)
//ss (有前导零的秒数)
fmt.Printf("当前时间:%s秒\n", now.Format("05"))
// (没有前导零的秒数)
fmt.Printf("当前时间:%s秒\n", now.Format("5"))
fmt.Printf("当前时间:%d秒\n", now.Second())
_, _, sec := now.Clock()
fmt.Printf("当前时间:%d秒\n", sec)
//YYYY-mm-dd HH:ii:ss
fmt.Printf("当前时间:%s\n", now.Format("2006-01-02 15:04:05"))
//YYYY/mm/dd HH:ii:ss
fmt.Printf("当前时间:%s\n", now.Format("2006/01/02 15:04:05"))
//获取指定日期的时间戳
date := time.Date(2015, 04, 01, 13, 11, 11, 0, time.Local)
fmt.Printf("%s的日期的时间戳:%d\n", date.Format("2006-01-02 15:04:05"), date.Unix())
//指定时区
prc := time.FixedZone("PRC", 8*3600) //注意第二个参数(基于UTC的秒数,8*3600为东八区)
date1 := time.Date(2015, 04, 01, 13, 11, 11, 0, prc)
fmt.Printf("%s的日期的时间戳:%d\n", date1.Format("2006-01-02 15:04:05"), date1.Unix())
newDate := date.Add(2 * time.Second)
fmt.Printf("%s两秒后的时间是:%s\n", date.Format("2006-01-02 15:04:05"), newDate.Format("2006-01-02 15:04:05"))
newDate = date.Add(-2 * time.Second)
fmt.Printf("%s两秒前的时间是:%s\n", date.Format("2006-01-02 15:04:05"), newDate.Format("2006-01-02 15:04:05"))
newDate = date.Add(-2 * time.Minute * 60 * 24)
fmt.Printf("%s两天前的时间是:%s\n", date.Format("2006-01-02 15:04:05"), newDate.Format("2006-01-02 00:00:00"))
newDate = date.AddDate(0, 0, -2)
fmt.Printf("%s两天前的时间是:%s\n", date.Format("2006-01-02 15:04:05"), newDate.Format("2006-01-02 00:00:00"))
//2015-04-01 13:11:11 到现在经过的秒数
fmt.Println(time.Now().Sub(date).Seconds())
fmt.Println(time.Since(date).Seconds())
if !time.Now().Before(date) {
fmt.Printf("%s 小于当前时间\n", date.Format("2006-01-02 15:04:05"))
}
if time.Now().After(date) {
fmt.Printf("%s 小于当前时间\n", date.Format("2006-01-02 15:04:05"))
}
if !time.Now().Equal(date) {
fmt.Printf("%s 不等于当前时间\n", date.Format("2006-01-02 15:04:05"))
}
fmt.Printf("今天是当年第%d天\n", now.YearDay())
fmt.Printf("今天是当月第%d日\n", now.Day())
//休眠3秒
time.Sleep(3 * time.Second)
}
文章源自编程技术分享-https://mervyn.life/c3ee5e76.html 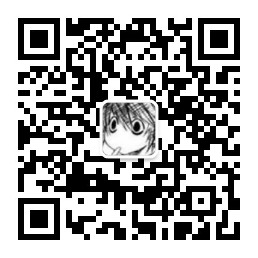
我的微信公众号
微信扫一扫
评论