目录
基础语法
变量
局部变量
通过 local
显示声明的为局部变量。局部变量的作用域为从声明位置开始到所在语句块结束。文章源自编程技术分享-https://mervyn.life/21d536c7.html
全局变量
变量全是全局变量,那怕是语句块或是函数里,除非用 local 显式声明为局部变量。文章源自编程技术分享-https://mervyn.life/21d536c7.html
注意: 变量的默认值均为 nil
文章源自编程技术分享-https://mervyn.life/21d536c7.html
对多个变量同时赋值,变量列表和值列表的各个元素用逗号分开,赋值语句右边的值会依次赋给左边的变量。文章源自编程技术分享-https://mervyn.life/21d536c7.html
a, b = 10, 2x --> a=10; b=2x
遇到赋值语句Lua会先计算右边所有的值然后再执行赋值操作,所以我们可以这样进行交换变量的值:文章源自编程技术分享-https://mervyn.life/21d536c7.html
x, y = y, x -- swap 'x' for 'y'
a[i], a[j] = a[j], a[i] -- swap 'a[i]' for 'a[j]'
注释
单行注释
-- 单行注释
多行注释
--[[
多行注释
]]
表达式
Lua提供了以下几种运算符类型:文章源自编程技术分享-https://mervyn.life/21d536c7.html
算术运算符
算术运算符 | 说明 |
---|---|
+ | 加法 |
- | 减法 |
* | 乘法 |
/ | 除法 |
^ | 指数 |
% | 取模 |
print(1 + 2) -->打印 3
print(5 / 10) -->打印 0.5。 这是Lua不同于c语言的
print(5.0 / 10) -->打印 0.5。 浮点数相除的结果是浮点数
-- print(10 / 0) -->注意除数不能为0,计算的结果会出错
print(2 ^ 10) -->打印 1024。 求2的10次方
local num = 1357
print(num % 2) -->打印 1
print((num % 2) == 1) -->打印 true。 判断num是否为奇数
print((num % 5) == 0) -->打印 false。判断num是否能被5整数
关系运算符
关系运算符 | 说明 |
---|---|
< | 小于 |
> | 大于 |
<= | 小于等于 |
>= | 大于等于 |
== | 等于 |
~= | 不等于 |
print(1 < 2) -->打印 true
print(1 == 2) -->打印 false
print(1 ~= 2) -->打印 true
local a, b = true, false
print(a == b) -->打印 false
注意:Lua 语言中“不等于”运算符的写法为:~=
文章源自编程技术分享-https://mervyn.life/21d536c7.html
逻辑运算符
逻辑运算符 | 说明 |
---|---|
and | 逻辑与 |
or | 逻辑或 |
not | 逻辑非 |
lua 中 and
和 or
比较特殊,规则如下:文章源自编程技术分享-https://mervyn.life/21d536c7.html
- a and b` 如果 a 为 nil,则返回 a,否则返回 b;
a or b
如果 a 为 nil,则返回 b,否则返回 a。
注意:所有逻辑操作符将 false 和 nil 视作假,其他任何值视作真,对于 and 和 or,“短路求值”,对于 not,永远只返回 true 或者 false。文章源自编程技术分享-https://mervyn.life/21d536c7.html
local c = nil
local d = 0
local e = 100
print(c and d) -->打印 nil
print(c and e) -->打印 nil
print(d and e) -->打印 100
print(c or d) -->打印 0
print(c or e) -->打印 100
print(not c) -->打印 true
print(not d) -->打印 false
其他运算符
数据类型
lua 支持的数据类型有:nil
number
string
boolean
table
function
userdata
thread
。文章源自编程技术分享-https://mervyn.life/21d536c7.html
函数 type()
能够返回一个值或一个变量所属的类型。文章源自编程技术分享-https://mervyn.life/21d536c7.html
print(type("Hello world")) --> string
print(type(10.4*3)) --> number
print(type(print)) --> function
print(type(type)) --> function
print(type(true)) --> boolean
print(type(nil)) --> nil
print(type(type(X))) --> string
nil
Lua将 nil
表示”无效值“。一个变量在第一次赋值前的默认值是 nil
文章源自编程技术分享-https://mervyn.life/21d536c7.html
local num
print(num) --nil
type(X) --nil
nil
作比较时应该加上双引号 "
,例:文章源自编程技术分享-https://mervyn.life/21d536c7.html
type(X) == nil -- 结果: false
type(X) == "nil" -- 结果: true
boolean
布尔类型,可选值 true
false
文章源自编程技术分享-https://mervyn.life/21d536c7.html
Lua 中 nil
和 false
为 “假”,其它所有值均为 true
。比如 0 和空字符串就是 “真”文章源自编程技术分享-https://mervyn.life/21d536c7.html
number
一般地,Lua 的 number
类型就是用双精度浮点数来实现的文章源自编程技术分享-https://mervyn.life/21d536c7.html
以下几种写法都被看作是 number 类型:文章源自编程技术分享-https://mervyn.life/21d536c7.html
print(type(2))
print(type(2.2))
print(type(0.2))
print(type(2e+1))
print(type(0.2e-1))
print(type(7.8263692594256e-06))
字符串
Lua 中有三种方式表示字符串:文章源自编程技术分享-https://mervyn.life/21d536c7.html
- 使用一对匹配的单引号。
str = 'this is string'
- 使用一对匹配的双引号。
str = "this is string"
- 字符串还可以用一种长括号(即
[[ ]]
)括起来的方式定义。
str = [[this is string\n]] -- output: this is string\n
str1 = [=[This is string.]=] -- output: This is string.
字符串连接
在 Lua 中连接两个字符串,可以使用操作符 ..
(两个点)。如果其任意一个操作数是数字的话,Lua 会将这个数字转换成字符串。注意,连接操作符只会创建一个新字符串,而不会改变原操作数。也可以使用 string 库函数 string.format
连接字符串。文章源自编程技术分享-https://mervyn.life/21d536c7.html
print("Hello " .. "World") -->打印 Hello World
print(0 .. 1) -->打印 01
str2 = string.format("%d-%s-%.2f",123,"world",1.21)
print(str2) -->打印 123-world-1.21
注意 如果有很多这样的连接操作(比如在循环中使用 .. 来拼接最终结果),则性能损耗会非常大。这种情况更推荐使用 table
和 table.concat()
。例:文章源自编程技术分享-https://mervyn.life/21d536c7.html
local pieces = {}
for i, elem in ipairs(my_list) do
pieces[i] = my_process(elem)
end
local res = table.concat(pieces)
table
table
类似 PHP 的关联数组。对 table 的索引使用方括号 []
。Lua 也提供了 .
操作文章源自编程技术分享-https://mervyn.life/21d536c7.html
- 创建一个空的table
local t1 = {}
- 直接初始化表
local enterprise = {
website = "www.mervyntang.com",
staff = {"Mervyn", "Jack"},
10086,
[4] = 100,
["address"] = "Shenzhen"
}
print(enterprise.website) --output: www.mervyntang.com
print(enterprise["website"]) --output: www.mervyntang.com
print(enterprise[website]) --output: nil
print(enterprise.staff[1]) --output: Mervyn
print(enterprise[0]) --output: nil
print(enterprise[1]) --output: 10086
print(enterprise[4]) --output: 100
print(enterprise["address"]) --output: Shenzhen
print(enterprise.address) --output: Shenzhen
- 动态构建表
a = {}
a["key"] = "value"
key = 10
a[key] = 22
a[key] = a[key] + 11
for k, v in pairs(a) do
print(k .. " : " .. v)
end
-- 执行结果:
-- key : value
-- 10 : 33
a3 = {}
for i = 1, 10 do
a3[i] = i
end
a3["key"] = "val"
print(a3["key"]) --执行结果:val
print(a3["none"]) --执行结果:nil
注意 表的默认索引从 1
开始。table 不会固定长度大小,有新数据添加时 table
长度会自动增长,没初始的 table 都是 nil。文章源自编程技术分享-https://mervyn.life/21d536c7.html
function
函数 也是一种数据类型,函数可以存储在变量中,可以通过参数传递给其他函数,还可以作为其他函数的返回值。文章源自编程技术分享-https://mervyn.life/21d536c7.html
function factorial1(n)
if n == 0 then
return 1
else
return n * factorial1(n - 1)
end
end
print(factorial1(5))
-- 把函数赋给变量
factorial2 = factorial1
print(factorial2(5))
local function func1()
end
-- 等价于
local func1 = function ()
end
控制结构
if / else
if condition then
-- do sth
elseif
-- do sth
else
-- do sth
end
注意: elseif
是连起来的,如果变为 else if
则相当于在 else 里嵌套另一个 if 语句文章源自编程技术分享-https://mervyn.life/21d536c7.html
if condition then
-- do sth
elseif
-- do sth
else
if condition then
-- do sth
else
-- do sth
end
end
while
while 表达式 do
-- do sth
end
当表达式值为假(即 false
或 nil
)时结束循环。文章源自编程技术分享-https://mervyn.life/21d536c7.html
repeat
执行 repeat 循环体后,直到 until 的条件为真时才结束文章源自编程技术分享-https://mervyn.life/21d536c7.html
例:文章源自编程技术分享-https://mervyn.life/21d536c7.html
x = 10
repeat
print(x)
until false
该代码将导致死循环,因为until的条件一直为假,循环不会结束文章源自编程技术分享-https://mervyn.life/21d536c7.html
for
for 语句有两种形式:数字 for(numeric for)和范型 for(generic for)。文章源自编程技术分享-https://mervyn.life/21d536c7.html
for 数字型
for var = begin, finish, step do
--body
end
for 泛型
-- 打印数组a的所有值
local a = {"a", "b", "c", "d"}
for i, v in ipairs(a) do
print("index:", i, " value:", v)
end
-- output:
index: 1 value: a
index: 2 value: b
index: 3 value: c
index: 4 value: d
Lua 的基础库提供了 ipairs,这是一个用于遍历数组的迭代器函数。在每次循环中,i 会被赋予一个索引值,同时 v 被赋予一个对应于该索引的数组元素值。文章源自编程技术分享-https://mervyn.life/21d536c7.html
下面是另一个类似的示例,演示了如何遍历一个 table 中所有的 key文章源自编程技术分享-https://mervyn.life/21d536c7.html
-- 打印table t中所有的key
for k in pairs(t) do
print(k)
end
注意: Lua中是没有 continue
这个关键字的文章源自编程技术分享-https://mervyn.life/21d536c7.html
函数
函数定义
Lua 使用关键字 function
定义函数,语法如下:文章源自编程技术分享-https://mervyn.life/21d536c7.html
function funcName (args) -- args 表示参数列表,函数的参数列表可以为空
-- body
end
上面的语法定义了一个全局函数,名为 function_name
. 全局函数本质上就是函数类型的值赋给了一个全局变量,即上面的语法等价于文章源自编程技术分享-https://mervyn.life/21d536c7.html
funcName = function (args)
-- body
end
我们应当尽量使用“局部函数”,只需要开头加上 local
修饰符:文章源自编程技术分享-https://mervyn.life/21d536c7.html
local function funcName (args)
-- body
end
注意: 函数的定义要放在函数调用之前。文章源自编程技术分享-https://mervyn.life/21d536c7.html
函数的参数
在调用函数的时候,若形参个数和实参个数不同时,Lua 会自动调整实参个数。调整规则:若实参个数大于形参个数,从左向右,多余的实参被忽略;若实参个数小于形参个数,从左向右,没有被实参初始化的形参会被初始化为 nil。文章源自编程技术分享-https://mervyn.life/21d536c7.html
local function fun1(a, b) --两个形参,多余的实参被忽略掉
print(a, b)
end
local function fun2(a, b, c, d) --四个形参,没有被实参初始化的形参,用nil初始化
print(a, b, c, d)
end
local x = 1
local y = 2
local z = 3
fun1(x, y, z) -- z被函数fun1忽略掉了,参数变成 x, y
fun2(x, y, z) -- 后面自动加上一个nil,参数变成 x, y, z, nil
-->output
1 2
1 2 3 nil
变长参数
若形参为 ...
, 表示该函数可以接收不同长度的参数。访问参数的时候也要使用 ...
。文章源自编程技术分享-https://mervyn.life/21d536c7.html
local function func( ... ) -- 形参为 ... ,表示函数采用变长参数
local temp = {...} -- 访问的时候也要使用 ...
local ans = table.concat(temp, " ") -- 使用 table.concat 库函数对数
-- 组内容使用 " " 拼接成字符串。
print(ans)
end
func(1, 2) -- 传递了两个参数
func(1, 2, 3, 4) -- 传递了四个参数
-->output
1 2
1 2 3 4
按引用传值
当函数参数是 table
类型时,传递进来的是 实际参数的引用,此时在函数内部对该 table 所做的修改,会直接对调用者所传递的实际参数生效,而无需自己返回结果和让调用者进行赋值。文章源自编程技术分享-https://mervyn.life/21d536c7.html
常用基本类型中,除了 table 是按址传递类型外,其它的都是按值传递参数。文章源自编程技术分享-https://mervyn.life/21d536c7.html
函数返回值
lua
允许函数返回多个值。返回多个值时,值之间用 ,
隔开。文章源自编程技术分享-https://mervyn.life/21d536c7.html
若返回值个数大于接收变量的个数,多余的返回值会被忽略掉; 若返回值个数小于参数个数,从左向右,没有被返回值初始化的变量会被初始化为 nil。文章源自编程技术分享-https://mervyn.life/21d536c7.html
function init() --init 函数 返回两个值 1 和 "lua"
return 1, "lua"
end
x = init()
print(x)
x, y, z = init()
print(x, y, z)
--output
1
1 lua nil
当一个函数有一个以上返回值,且函数调用不是一个列表表达式的最后一个元素,那么函数调用只会产生一个返回值, 也就是第一个返回值。文章源自编程技术分享-https://mervyn.life/21d536c7.html
local function init() -- init 函数 返回两个值 1 和 "lua"
return 1, "lua"
end
local x, y, z = init(), 2 -- init 函数的位置不在最后,此时只返回 1
print(x, y, z) -->output 1 2 nil
local a, b, c = 2, init() -- init 函数的位置在最后,此时返回 1 和 "lua"
print(a, b, c) -->output 2 1 lua
如果你确保只取函数返回值的第一个值,可以使用括号运算符,例如文章源自编程技术分享-https://mervyn.life/21d536c7.html
local function init()
return 1, "lua"
end
print((init()), 2) -->output 1 2
print(2, (init())) -->output 2 1
常用库、函数
字符串函数
-- 字符串操作
string.upper(argument) -- 字符串全部转为大写字母。
string.lower(argument) -- 字符串全部转为小写字母。
string.gsub(mainString,findString,replaceString,num) -- 在字符串中替换,mainString为要替换的字符串, findString 为被替换的字符,replaceString 要替换的字符,num 替换次数(可以忽略,则全部替换)
string.find (str, substr, [init, [end]]) -- 在一个指定的目标字符串中搜索指定的内容(第三个参数为索引),返回其具体位置。不存在则返回 nil。
string.reverse(arg) -- 字符串反转
string.format(...) -- 返回一个类似printf的格式化字符串
string.char(arg) -- char 将整型数字转成字符并连接
string.byte(arg[,int]) -- byte 转换字符为整数值(可以指定某个字符,默认第一个字符)。
string.len(arg) -- 计算字符串长度
string.rep(string, n) -- 返回字符串string的n个拷贝
string.gmatch(str, pattern) -- 回一个迭代器函数,每一次调用这个函数, 返回一个在字符串 str 找到的下一个符合 pattern 描述的子串。如果参数 pattern 描述的字符串没有找到,迭代函数返回nil。
string.match(str, pattern, init) -- 只寻找源字串str中的第一个配对. 参数init可选, 指定搜寻过程的起点, 默认为1。在成功配对时, 函数将返回配对表达式中的所有捕获结果; 如果没有设置捕获标记, 则返回整个配对字符串. 当没有成功的配对时, 返回nil。
string.upper(str) -- 如何对字符串大小写进行转换
string.lower(str)
string.find(string, substr) -- 对字符串进行查找与反转操作
-- 示例
string.gsub("aaaa","a","z",3) -- zzza3
string.find("Hello Lua user", "Lua", 1) -- 7 9
string.reverse("Lua") -- auL
string.format("the value is:%d",4) -- the value is:4
string.char(97,98,99,100) -- abcd
string.byte("ABCD",4) -- 68
string.len("abc") -- 3
string.rep("abcd",2) -- abcdabcd
for word in string.gmatch("Hello Lua user", "%a+") do print(word) end -- 输出: Hello Lua user
string.match("I have 2 questions for you.", "%d+ %a+") -- 2 questions
string.format("%d, %q", string.match("I have 2 questions for you.", "(%d+) (%a+)")) -- 2, "questions"
print(string.upper("Lua")) -- LUA
string.lower("Lua") -- lua
string1 = "Lua"
string2 = "Tutorial"
number1 = 10
number2 = 20
-- 基本字符串格式化
print(string.format("基本格式化 %s %s",string1,string2))
-- 日期格式化
date = 2; month = 1; year = 2014
print(string.format("日期格式化 %02d/%02d/%03d", date, month, year))
-- 十进制格式化
print(string.format("%.4f",1/3))
string.format("%c", 83) -- 输出S
string.format("%+d", 17.0) -- 输出+17
string.format("%05d", 17) -- 输出00017
string.format("%o", 17) -- 输出21
string.format("%u", 3.14) -- 输出3
string.format("%x", 13) -- 输出d
string.format("%X", 13) -- 输出D
string.format("%e", 1000) -- 输出1.000000e+03
string.format("%E", 1000) -- 输出1.000000E+03
string.format("%6.3f", 13) -- 输出13.000
string.format("%q", "One\nTwo") -- 输出"One\
-- Two"
string.format("%s", "monkey") -- 输出monkey
string.format("%10s", "monkey") -- 输出 monkey
string.format("%5.3s", "monkey") -- 输出 mon
时间函数
os.time ([table]) -- 返回当前的时间和日期(它表示从某一时刻到现在的秒数)。如果用 table 参数,它会返回一个数字,表示该 table 中 所描述的日期和时间(它表示从某一时刻到 table 中描述日期和时间的秒数)。
--[[
table 的字段如下:
year 四位数字
month 1--12
day 1--31
hour 0--23
min 0--59
sec 0--61
isdst boolean(true表示夏令时)
格式字符与含义:
%a 一星期中天数的简写(例如:Wed)
%A 一星期中天数的全称(例如:Wednesday)
%b 月份的简写(例如:Sep)
%B 月份的全称(例如:September)
%c 日期和时间(例如:07/30/15 16:57:24)
%d 一个月中的第几天[01 ~ 31]
%H 24小时制中的小时数[00 ~ 23]
%I 12小时制中的小时数[01 ~ 12]
%j 一年中的第几天[001 ~ 366]
%M 分钟数[00 ~ 59]
%m 月份数[01 ~ 12]
%p “上午(am)”或“下午(pm)”
%S 秒数[00 ~ 59]
%w 一星期中的第几天[1 ~ 7 = 星期天 ~ 星期六]
%x 日期(例如:07/30/15)
%X 时间(例如:16:57:24)
%y 两位数的年份[00 ~ 99]
%Y 完整的年份(例如:2015)
%% 字符'%'
]]
os.difftime (t2, t1) -- 返回 t1 到 t2 的时间差,单位为秒。
os.date ([format [, time]]) -- 把一个表示日期和时间的数值,转换成更高级的表现形式。
-- 其第一个参数 format 是一个格式化字符串,描述了要返回的时间形式。
-- 第二个参数 time 就是日期和时间的数字表示,缺省时默认为当前的时间。
-- 使用格式字符 "*t",创建一个时间表。
print(os.time()) -->output 1438243393
local t1 = { year = 1970, month = 1, day = 1, hour = 8, min = 1 }
print(os.time(t1)) -->output 60
local day2 = { year = 2015, month = 7, day = 31 }
local t2 = os.time(day2)
print(os.difftime(t2, t1)) -->output 86400
local tab1 = os.date("*t") --返回一个描述当前日期和时间的表
local ans1 = "{"
for k, v in pairs(tab1) do --把tab1转换成一个字符串
ans1 = string.format("%s %s = %s,", ans1, k, tostring(v))
end
ans1 = ans1 .. "}"
print("tab1 = ", ans1)
-->output
tab1 = { hour = 17, min = 28, wday = 5, day = 30, month = 7, year = 2015, sec = 10, yday = 211, isdst = false,}
tab2 = { hour = 8, min = 6, wday = 5, day = 1, month = 1, year = 1970, sec = 0, yday = 1, isdst = false,}
print(os.date("today is %A, in %B")) -- today is Thursday, in July
print(os.date("now is %x %X")) -- now is 07/30/15 17:39:22
数学库
math.rad(x) -- 角度x转换成弧度
math.deg(x) -- 弧度x转换成角度
math.max(x, ...) -- 返回参数中值最大的那个数,参数必须是number型
math.min(x, ...) -- 返回参数中值最小的那个数,参数必须是number型
math.random ([m [, n]]) -- 不传入参数时,返回 一个在区间[0,1)内均匀分布的伪随机实数;
-- 只使用一个整数参数m时,返回一个在区间[1, m]内均匀分布的伪随机整数;
-- 使用两个整数参数时,返回一个在区间[m, n]内均匀分布的伪随机整数
math.randomseed (x) -- 为伪随机数生成器设置一个种子x,相同的种子将会生成相同的数字序列
math.abs(x) -- 返回x的绝对值
math.fmod(x, y) -- 返回 x对y取余数
math.pow(x, y) -- 返回x的y次方
math.sqrt(x) -- 返回x的算术平方根
math.exp(x) -- 返回自然数e的x次方
math.log(x) -- 返回x的自然对数
math.log10(x) -- 返回以10为底,x的对数
math.floor(x) -- 返回最大且不大于x的整数
math.ceil(x) -- 返回最小且不小于x的整数
math.pi -- 圆周率
math.sin(x) -- 求弧度x的正弦值
math.cos(x) -- 求弧度x的余弦值
math.tan(x) -- 求弧度x的正切值
math.asin(x) -- 求x的反正弦值
math.acos(x) -- 求x的反余弦值
math.atan(x) -- 求x的反正切值
文件操作
--[[
模式:
模式 含义 文件不存在时
"r" 读模式 (默认) 返回nil加错误信息
"w" 写模式 创建文件
"a" 添加模式 创建文件
"r+" 更新模式,保存之前的数据 返回nil加错误信息
"w+" 更新模式,清除之前的数据 创建文件
"a+" 添加更新模式,保存之前的数据,在文件尾进行添加 创建文件
]]
io.open (filename [, mode]) -- 按指定的模式 mode,打开一个文件名为 filename 的文件,成功则返回文件句柄,失败则返回 nil 加错误信息。
io.close () -- 关闭文件,和 file:close() 的作用相同。没有参数 file 时,关闭默认输出文件。
io.lines ([filename]) -- 打开指定的文件 filename 为读模式并返回一个迭代函数
io.output () -- 类似于 io.input,但操作在默认输出文件上。
io.read (...) -- 相当于 io.input():read
io.type (obj) -- 检测 obj 是否一个可用的文件句柄。
io.write (...) -- 相当于 io.output():write。
file:read (...) -- 按指定的格式读取一个文件。
file:close () -- 关闭文件。注意:当文件句柄被垃圾收集后,文件将自动关闭。句柄将变为一个不可预知的值。
file:write (...) -- 把每一个参数的值写入文件。
-- 参数必须为字符串或数字,若要输出其它值,则需通过 tostring 或 string.format 进行转换。
file:seek ([whence] [, offset]) -- 设置和获取当前文件位置,成功则返回最终的文件位置(按字节,相对于文件开头) 失败则返回 nil 加错误信息。
-- 缺省时,whence 默认为 "cur",offset 默认为 0 。 参数 whence:
-- whence 含义
-- "set" 文件开始
-- "cur" 文件当前位置(默认)
-- "end" 文件结束
file:setvbuf (mode [, size]) -- 设置输出文件的缓冲模式
-- 模式 含义
-- "no" 没有缓冲,即直接输出
-- "full" 全缓冲,即当缓冲满后才进行输出操作(也可调用flush马上输出)
-- "line" 以行为单位,进行输出
-- 最后两种模式,size 可以指定缓冲的大小(按字节),忽略 size 将自动调整为最佳的大小。
I/O 库
Lua I/O 库提供两种不同的方式处理文件:隐式文件描述
显式文件描述
文章源自编程技术分享-https://mervyn.life/21d536c7.html
-- 隐式文件描述:
-- 打开已经存在的 test1.txt 文件,并读取里面的内容
file = io.input("test1.txt") -- 使用 io.input() 函数打开文件
repeat
line = io.read() -- 逐行读取内容,文件结束时返回nil
if nil == line then
break
end
print(line)
until (false)
io.close(file) -- 关闭文件
-- 在 test1.txt 文件的最后添加一行 "hello world"
file = io.open("test1.txt", "a+") -- 使用 io.open() 函数,以添加模式打开文件
io.output(file) -- 使用 io.output() 函数,设置默认输出文件
io.write("\nhello world") -- 使用 io.write() 函数,把内容写到文件
io.close(file)
-- 显式文件描述
-- 使用 file:XXX() 函数方式进行操作, 其中 file 为 io.open() 返回的文件句柄。
-- 打开已经存在的 test2.txt 文件,并读取里面的内容
file = io.open("test2.txt", "r") -- 使用 io.open() 函数,以只读模式打开文件
for line in file:lines() do -- 使用 file:lines() 函数逐行读取文件
print(line)
end
file:close()
-- 在 test2.txt 文件的最后添加一行 "hello world"
file = io.open("test2.txt", "a") -- 使用 io.open() 函数,以添加模式打开文件
file:write("\nhello world") -- 使用 file:write() 函数,在文件末尾追加内容
file:close()
文章源自编程技术分享-https://mervyn.life/21d536c7.html 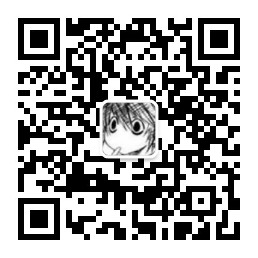
评论