目录
创建工程环境
-
sass创建文章源自编程技术分享-https://mervyn.life/102e9495.html
直接创建目录即可
文章源自编程技术分享-https://mervyn.life/102e9495.html -
compass 创建文章源自编程技术分享-https://mervyn.life/102e9495.html
-
cd xxx_dir文章源自编程技术分享-https://mervyn.life/102e9495.html
-
compass create文章源自编程技术分享-https://mervyn.life/102e9495.html
-
compass create --bare
--sass-dir "sass"
--css-dir "css"
--images-dir "images"
--javascript-dir "js"`
编译文件
- sass编译
sass xxx.scss xxx.css
也可以指定输出css文件的格式文章源自编程技术分享-https://mervyn.life/102e9495.html
sass xxx.scss xxx.css --style = nested | expanded | compact | compressed
监视sass/scss文件变化文章源自编程技术分享-https://mervyn.life/102e9495.html
sass --watch xxx.scss:xxx.css --style=compact
监视目录变化文章源自编程技术分享-https://mervyn.life/102e9495.html
sass --watch sass_dir:css_dir
-
compass编译文章源自编程技术分享-https://mervyn.life/102e9495.html
需要理解config.rb文件的配置,因为compass编译基于compass的config.rb文件内容文章源自编程技术分享-https://mervyn.life/102e9495.html
compass compile // 只会编译有变化的sass文件
如果要强制编译所有的sass文件,可以用文章源自编程技术分享-https://mervyn.life/102e9495.html
compass compile --force
监视文件变化文章源自编程技术分享-https://mervyn.life/102e9495.html
compass watch
compass watch --force
语法相关
sass文件注释
-
单行注释文章源自编程技术分享-https://mervyn.life/102e9495.html
// 不会编译进css文件文章源自编程技术分享-https://mervyn.life/102e9495.html
-
标准注释
/* */
会编译进css文件,压缩模式不会编译进css文件文章源自编程技术分享-https://mervyn.life/102e9495.html -
重要注释
/*! xxx */
重要注释,压缩模式也会编译进css文件文章源自编程技术分享-https://mervyn.life/102e9495.html变量
变量定义
$开头
,如 类似 $color文章源自编程技术分享-https://mervyn.life/102e9495.html
例:文章源自编程技术分享-https://mervyn.life/102e9495.html
body {
$color : red; //局部变量定义
color: $color;
}
font {
color:$color; //这个时候会提示Error: Undefined variable: "$color".,因为上边定义的变量只在body 代码块内生效。
}
- 全局变量
变量定义后加 !global
如:文章源自编程技术分享-https://mervyn.life/102e9495.html
body {
$color: red !global; //全局变量定义
background: $color;
}
footer {
background:$color;
}
- 变量默认值
$size: 10px; //第一次为变量定义
$size: 12px; //第二次为重新赋值
body {
font-size: $size;
}
编译后对应的css文章源自编程技术分享-https://mervyn.life/102e9495.html
body {
font-size: 12px;
}
- !default 用法
$size: 10px; //此时为重新赋值
$size: 12px !default; //优先以它为变量定义
body {
font-size: $size;
}
编译后对应的css文章源自编程技术分享-https://mervyn.life/102e9495.html
body {
font-size: 10px;
}
-
多值变量
变量有多个值,List类型和Map类型文章源自编程技术分享-https://mervyn.life/102e9495.html$margins: 1px 2px 3px 4px; //List类型 $maps: (color_1:red, font_1:blue); //Map类型
-
变量特殊用法文章源自编程技术分享-https://mervyn.life/102e9495.html
可以用在属性或者选择器上,使用
#{变量名}
文章源自编程技术分享-https://mervyn.life/102e9495.html
例:文章源自编程技术分享-https://mervyn.life/102e9495.html
$margins: 1px 2px 3px 4px; //List类型
$maps: (color_1:red, font_1:blue); //Map类型
$className: main;
body {
margin: $margins;
margin-left: nth($margins, 1);
}
footer {
background-color: map-get($maps, color_1);
font:map-get($maps, font_1);
}
//可以用在属性或者选择器上,使用#{变量名}
.#{$className}{
background-color: red;
}
##{$className} {
background-color: green;
}
//变量用中横线和下划线是同样的变量
$size_1 : 12px;
$size-1: 13px;
header {
font: $size_1;
}
编译后对应的css文章源自编程技术分享-https://mervyn.life/102e9495.html
body {
margin: 1px 2px 3px 4px;
margin-left: 1px;
}
footer {
background-color: red;
font: blue;
}
.main {
background-color: red;
}
#main {
background-color: green;
}
header {
font: 13px;
}
数据类型
Number/String/List/Map/Color/Boolean/Null文章源自编程技术分享-https://mervyn.life/102e9495.html
// 数字类型
$n1: 1.2;
$n2: 2;
$n3: 12px;
p {
font-size: $n3;
}
//字符串类型
$s1: container;
$s2: 'container';
$s3: "container";
.#{$s3} {
font-size: $n3;
}
//Boolean类型
$bt: true;
$bf: false;
// Null类型
$null: null;
//Color类型
$c1: blue;
$c2: #ffeedd;
$c3: rgba(255,255,0, 0.5);
body {
color: $c3;
}
部分文件
以下划线开头的文件,不会被编译成css文件
如 _font.scss
文件文章源自编程技术分享-https://mervyn.life/102e9495.html
样式导入文章源自编程技术分享-https://mervyn.life/102e9495.html
- 被导入的文件的名字 以 .css结尾
- 被导入的文件的名字是个url地址(比如:http://xxxx/xxx.css)
3.被导入的文件的名字是css的url()的值
以上3种情况会被识别成原生的css导入文章源自编程技术分享-https://mervyn.life/102e9495.html
例:
_part.scss
文章源自编程技术分享-https://mervyn.life/102e9495.html
header {
font-family: 微软雅黑
}
demo.scss文章源自编程技术分享-https://mervyn.life/102e9495.html
@import url(xx.css);
@import 'http://wwwx.xxx';
@import 'xx.css';
@import "part"; //或者用以下两种方式
// @import "_part";
// @import "_part.scss";
编译成的 demo.css文章源自编程技术分享-https://mervyn.life/102e9495.html
@charset "UTF-8";
@import url(xx.css);
@import "http://wwwx.xxx";
@import url(xx.css);
header {
font-family: 微软雅黑;
}
嵌套
body {
background: red;
// 选择器嵌套
header {
background: green;
}
//属性嵌套, 注意冒号
footer {
background :{
color : blue;
size : 12px;
}
}
a {
color :green;
//引用父选择器
&:hover {
color: yellow;
}
//注意以下区别
.content {
background: red;
}
&.content {
background: green;
}
}
//@at-root 跳出嵌套
@at-root .container {
width: 1104px;
}
@media screen and (max-width:600px) {
@at-root(without: media) {
.container {
background: red;
}
}
@at-root(without: media rule) {
.container {
background: red;
}
}
}
// @at-root 和 & 结合使用
.row {
color : red;
@at-root nav & {
color : blue;
}
}
}
编译后的css文章源自编程技术分享-https://mervyn.life/102e9495.html
body {
background: red;
}
body header {
background: green;
}
body footer {
background-color: blue;
background-size: 12px;
}
body a {
color: green;
}
body a:hover {
color: yellow;
}
body a .content {
background: red;
}
body a.content {
background: green;
}
.container {
width: 1104px;
}
body .container {
background: red;
}
.container {
background: red;
}
body .row {
color: red;
}
nav body .row {
color: blue;
}
默认@at-root
只会跳出选择器嵌套,不能跳出 @media
或@support
,如果要跳出这两种,则需使用@at-root(without: media)
, @at-root(without:support)
.这个语法的关键词有四个 :文章源自编程技术分享-https://mervyn.life/102e9495.html
all 表示所有
rule 表示常规css
media 表示media
默认的@at-root 其实就是@at-root(without: rule)文章源自编程技术分享-https://mervyn.life/102e9495.html
继承
.alert {
background-color: #ffeedd;
}
.small {
font-size: 12px;
}
//继承与多继承
.alert-info {
// @extend .alert;
// @extend .small;
//或者使用
@extend .alert, .small;
color: red;
}
//链式继承
.one {
border: 1px solid red;
}
.two {
@extend .one;
color : red;
}
.three {
@extend .two;
color: green;
}
编译后的css文章源自编程技术分享-https://mervyn.life/102e9495.html
.alert, .alert-info {
background-color: #ffeedd;
}
.small, .alert-info {
font-size: 12px;
}
.alert-info {
color: red;
}
.one, .two, .three {
border: 1px solid red;
}
.two, .three {
color: red;
}
.three {
color: green;
}
虽然能继承的选择器数量很多,但也有很多是不能被支持的。如包含选择器(.one .two) 或者是相邻兄弟选择器(.one+.two)文章源自编程技术分享-https://mervyn.life/102e9495.html
如果继承的元素是a, 同时 a有hover状态的形式,那么hover 也将被继承文章源自编程技术分享-https://mervyn.life/102e9495.html
-
交叉继承 (尽量避免)文章源自编程技术分享-https://mervyn.life/102e9495.html
a span { font-size: 12px; }
div .content {
@extend span;
}文章源自编程技术分享-https://mervyn.life/102e9495.html
css
```css
a span, a div .content, div a .content {
font-size: 12px;
}
占位选择器
%开头
来定义选择器,此选择器的样式不会被编译进css文件文章源自编程技术分享-https://mervyn.life/102e9495.html
%alert {
background-color: #ffeedd;
}
.small {
font-size: 12px;
}
//继承与多继承
.alert-info {
// @extend .alert;
// @extend .small;
//或者使用
@extend %alert, .small;
color: red;
}
css文章源自编程技术分享-https://mervyn.life/102e9495.html
.alert-info {
background-color: #ffeedd;
}
.small, .alert-info {
font-size: 12px;
}
.alert-info {
color: red;
}
条件控制
@if
//@if 的用法
$screenWidth: 800;
body {
color: if($screenWidth > 768, blue, red)
}
@if $screenWidth > 768 {
body {
color: red;
}
} @elseif $screenWidth > 400 {
span {
color: yellow;
}
} @else {
p {
color: blue;
}
}
循环
for 的用法
@for $i from 1 through 5 {
.span#{$i} {
width: 20% * $i;
}
}
@for $i from 1 to 5 {
.span#{$i} {
width: 20% * $i;
}
}
while 用法
$j: 6;
@while $j > 0 {
.p#{$j} {
width: 20% * $j;
}
$j: $j - 3;
}
eash 常规遍历
$k: 1;
@each $c in blue, red, yellow {
.div#{$k} {
color: $c;
}
$k: $k + 1
}
//eash List遍历
@each $key, $color in (defalut, blue), (info, green), (danger, red) {
.text-#{$key} {
color: $color;
}
}
//eash Map遍历
@each $key, $value in (defalut: blue, info: green, danger: red) {
.label-#{$key} {
color: $value;
}
}
@mixin
//代码片段
@mixin func_1 {
color: red;
}
body {
@include func_1;
}
//带参数的片段
@mixin func_2($color: red) {
color: $color;
}
body {
@include func_2(#fff);
}
//带多个参数的片段
@mixin func_3($color: red, $font-size: 14px) {
color: $color;
font-size: $font-size;
}
body {
@include func_3($font-size: 18px);
}
//未知参数个数的片段
@mixin func_4($shadow...) {
-moz-box-shadow: $shadow;
-webkit-box-shadow: $shadow;
box-shadow:$shadow;
}
.shadows {
@include func_4(0px 4px 4px #555, 2px 2px 2px #666);
}
函数与调试 (待完善)
创建一个项目
初始化
- npm init
- compass create --bare
创建基本框架
src
sass
- base
- components
- helpers
- layout
- pages
- themes
main.scss
config.rb
package.json
自动化构建
-
gulp构建工具文章源自编程技术分享-https://mervyn.life/102e9495.html
-
gulp-compass
www.npmjs.com/package/gulp-compasss文章源自编程技术分享-https://mervyn.life/102e9495.html -
browser-sync文章源自编程技术分享-https://mervyn.life/102e9495.html
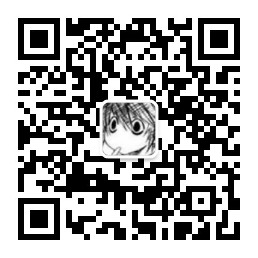
评论